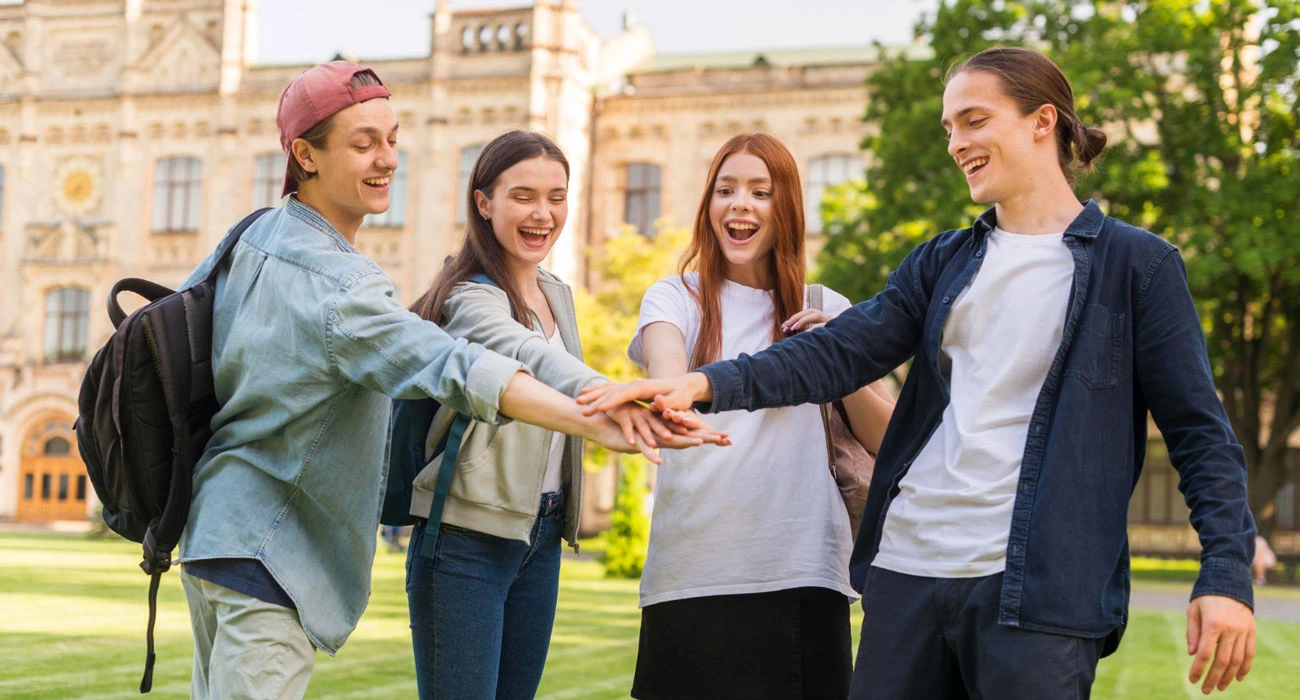
Automation Testing Framework Mastery
Course Description
Automation Testing Framework Mastery is a comprehensive course designed to help you develop a deep understanding of building robust, scalable, and maintainable automation testing frameworks.
- This course focuses on the creation of custom frameworks from scratch using Selenium WebDriver and TestNG with Java, providing you with the skills to design efficient test structures.
- You’ll learn to incorporate best practices such as data-driven, keyword-driven, and hybrid testing approaches, and integrate advanced features like report generation and continuous integration.
- Whether you’re new to automation or aiming to refine your framework-building skills, this course will guide you through the entire process of mastering automation frameworks.
What You’ll Learn From This Course
- Core concepts of automation testing frameworks
- Building a custom Selenium WebDriver framework with Java
- Implementing TestNG for test management and execution
- Design patterns for data-driven, keyword-driven, and hybrid frameworks
- Developing reusable page object models (POM)
- Creating advanced test reports with Extent Reports and Allure
- Integrating with CI/CD pipelines (Jenkins)
- Handling dynamic elements and wait strategies in Selenium
- Debugging and optimizing automation scripts
- Best practices for framework maintenance and scalability
Certification
Upon successfully completing this course, you will receive a Certificate of Completion in Automation Testing Framework Mastery. This certification highlights your ability to design, build, and maintain custom automation frameworks, showcasing your expertise in modern testing practices with industry-leading tools.
Learning Path
Section 1: Introduction to Automation Testing Frameworks
This section introduces the fundamentals of automation testing frameworks, their significance, and the different types of frameworks commonly used in software testing. Topics include:
- What is an automation testing framework?
- Importance of using frameworks in automation testing.
- Types of automation frameworks (Modular, Data-Driven, Keyword-Driven, Hybrid, etc.).
- Benefits of a well-structured framework.
- Overview of tools and technologies used in modern frameworks.
Section 2: Setting Up Your Development Environment
Learn how to set up the essential tools and environment for building and running automation frameworks. Topics include:
- Installing and configuring Java or other programming languages.
- Setting up IDEs (e.g., Eclipse, IntelliJ) for automation projects.
- Installing Selenium WebDriver and configuring it for use.
- Overview of Maven and Gradle for project management.
- Introduction to version control systems like Git.
- Setting up CI/CD pipelines for automated test execution.
Section 3: Core Concepts of Test Automation Frameworks
Understand the core concepts that are foundational to building effective automation frameworks. Topics include:
- What makes a good automation framework?
- Introduction to Page Object Model (POM) and its importance.
- Understanding locators and selector strategies (XPath, CSS).
- Implementing reusable functions and methods.
- Exception handling in test automation.
- Integrating external libraries for advanced functionalities.
Section 4: Building a Modular Automation Framework
In this section, you’ll learn to build a modular framework where test scripts are divided into independent modules. Topics include:
- Introduction to modular frameworks.
- Breaking down test cases into reusable modules.
- Creating independent test scripts for each module.
- Managing dependencies between modules.
- Designing test flow for modular test execution.
- Advantages and challenges of modular frameworks.
Section 5: Data-Driven Automation Framework Design
This section covers the design and implementation of data-driven frameworks where test data is separated from the test scripts. Topics include:
- What is a data-driven framework?
- Storing and managing test data (Excel, CSV, databases).
- Parameterizing test scripts with external data sources.
- Data-driven testing with Apache POI or other libraries.
- Implementing data providers in TestNG and JUnit.
- Validating different test scenarios with various data sets.
Section 6: Keyword-Driven Framework Development
Learn how to create a keyword-driven framework where test cases are written using keywords. Topics include:
- What is a keyword-driven framework?
- Creating keywords for actions and verifications.
- Designing a keyword-driven test script.
- Creating a keyword repository.
- Mapping keywords to functions in the automation code.
- Advantages and limitations of keyword-driven frameworks.
Section 7: Hybrid Framework Architecture
Explore the hybrid framework, which combines the best aspects of data-driven and keyword-driven approaches. Topics include:
- What is a hybrid automation framework?
- Combining modular, data-driven, and keyword-driven strategies.
- Structuring your test automation code for maximum flexibility.
- Using hybrid frameworks to support complex test scenarios.
- Implementing data-driven keyword execution.
- Best practices for maintaining a hybrid framework.
Section 8: Advanced Test Automation Framework Components
This section dives deeper into advanced concepts and components used in mature frameworks. Topics include:
- Implementing logging and reporting mechanisms.
- Integrating TestNG/JUnit for test execution and reporting.
- Using the Page Factory pattern in Page Object Model (POM).
- Advanced test suite management techniques.
- Building custom utilities for common actions (screenshots, wait conditions, etc.).
- Handling dynamic content and AJAX calls in web applications.
Section 9: Integrating Automation Frameworks with CI/CD Pipelines
Learn how to integrate your automation framework with CI/CD tools like Jenkins for continuous testing. Topics include:
- Overview of CI/CD in test automation.
- Setting up Jenkins for automated test execution.
- Running automated tests as part of the CI/CD pipeline.
- Integrating test frameworks with Docker and containerized environments.
- Generating reports and notifications after test execution.
- Best practices for scaling automated tests in CI/CD.
Section 10: Cross-Browser and Mobile Automation Frameworks
This section covers the implementation of frameworks that support cross-browser and mobile testing. Topics include:
- Setting up Selenium Grid for cross-browser testing.
- Configuring browser capabilities for different environments (Chrome, Firefox, Safari).
- Implementing mobile testing using Appium.
- Designing frameworks that support both web and mobile automation.
- Running tests on cloud platforms (BrowserStack, Sauce Labs).
- Cross-browser compatibility testing best practices.
Section 11: API Testing in Automation Frameworks
Learn how to integrate API testing into your automation framework. Topics include:
- Introduction to API testing with Rest Assured.
- Configuring API tests in a Selenium-based framework.
- Validating API responses and using assertions.
- Integrating API and UI tests for end-to-end testing.
- Storing and managing API test data.
- Reporting and logging for API test execution.
Section 12: Performance Testing Integration with Automation Frameworks
Understand how to extend your framework to support performance testing tools like JMeter. Topics include:
- Overview of performance testing and why it matters.
- Integrating JMeter with Selenium for hybrid tests.
- Running performance tests as part of CI pipelines.
- Analyzing performance metrics and reporting results.
- Using automation frameworks for load and stress testing.
- Best practices for combining functional and performance testing.
Section 13: Custom Reporting and Logging in Automation Frameworks
This section focuses on enhancing test reporting and logging mechanisms to gain better insights into test execution. Topics include:
- Generating detailed HTML and PDF reports for test execution.
- Customizing reports with test metrics, screenshots, and logs.
- Implementing real-time logging using Log4j or other libraries.
- Capturing screenshots for failed test cases.
- Creating dashboards for test results visualization.
- Integrating with external reporting tools like Allure or ExtentReports.
Section 14: Debugging and Maintaining Automation Frameworks
This section covers techniques for debugging and maintaining your automation frameworks. Topics include:
- Debugging automation scripts in IDEs (Eclipse, IntelliJ).
- Using breakpoints and stepping through code.
- Refactoring code for better maintainability.
- Handling deprecated or obsolete libraries.
- Managing large test suites and avoiding flaky tests.
- Strategies for framework updates and version control.
Section 15: Best Practices for Automation Framework Development
Learn industry best practices for designing, developing, and maintaining automation frameworks. Topics include:
- Writing clean and maintainable code for automation.
- Organizing test cases and test suites effectively.
- Ensuring scalability and reusability in your framework.
- Managing test data efficiently.
- Performance optimization tips for large-scale test executions.
- Keeping your framework adaptable to new technologies and updates.
Section 16: Final Project: Building a Robust Automation Framework
In this final section, you’ll apply everything you’ve learned to build a complete, real-world automation framework from scratch. Topics include:
- Defining the project scope and requirements.
- Designing the framework architecture.
- Implementing the core framework features.
- Running cross-browser, API, and performance tests.
- Generating detailed reports and integrating with CI/CD.
- Submitting the final framework project for evaluation.